As part of my job, I am required to learn how to use Spring. Thus, I will be going through this Pluralsight course, Spring Framework: Spring 5 Fundamentals by Bryan Hansen. I’m going into this blind with little to no knowledge of this framework. As the course I’m following is from 2019, my process will contain some differences from the material due to updates or new problems I may encounter. Your experience may vary as well if you’re following along my guide well after I’ve written it. (Hello, future reader!)
I will be dividing this course into multiple blog posts, starting with this post, What Is Spring? I will link the rest here as I complete them:
- What Is Spring?
- Architecture and Project Setup
- Spring Configuration Using Java
- Spring Scopes and Autowiring
- Spring Configuration Using XML
- Advanced Bean Configuration
Let’s spring into Spring, shall we? (heh)
What the Hell Is Spring?
Spring is a framework developed to reduce the complexities around enterprise Java development.
It later provided enterprise development without EJB (Enterprise Java Beans, which is a Java API used to develop robust and scalable distributed applications for businesses). While Spring can be used with or without Java Beans, it is now primarily used without them. This is significant as it allows us to do enterprise development without using an application server.
Spring is completely POJO (Plain Old Java Object) based. Any code you write in Spring can be used without using any Spring at all. The Spring Framework isn’t doing anything behind the scenes and just allows us to write better, cleaner code.
Spring was developed out of the frustrations of working with J2EE (Java 2 Platform, Enterprise Edition), thus it tries to be unobtrusive and should be easy to use.
Spring uses AOP (Aspect-Oriented Programming) and proxies to implement the function of cross-cutting concern. Cross-cutting concerns are aspects of a program that affect other concerns (a particular set of information that has an effect on the code). From my understanding, this means Spring supports modularity to prevent one piece of code from affecting another piece of code. In the end, your code should be smaller and more lightweight from using Spring.
Spring is built around best practices, which leads to us having design patterns (singletons, factories, abstract factories, etc.) in our code without us realizing that we’re using them. While we should know about these design patterns beforehand (which I totally don’t and only barely remember), Spring allows less experienced developers to use them effectively without having the knowledge firsthand.
The Problem
What problem is Spring trying to solve? Well Spring can help us do the following things:
- Increase testability
- Increase code maintainability
- Increase scalability
- Reduce code complexity
- Allow us to focus on business instead of coding
Business Focus
Let’s take a look at the following code, which was written with the help of JDBC (Java Database Connectivity).
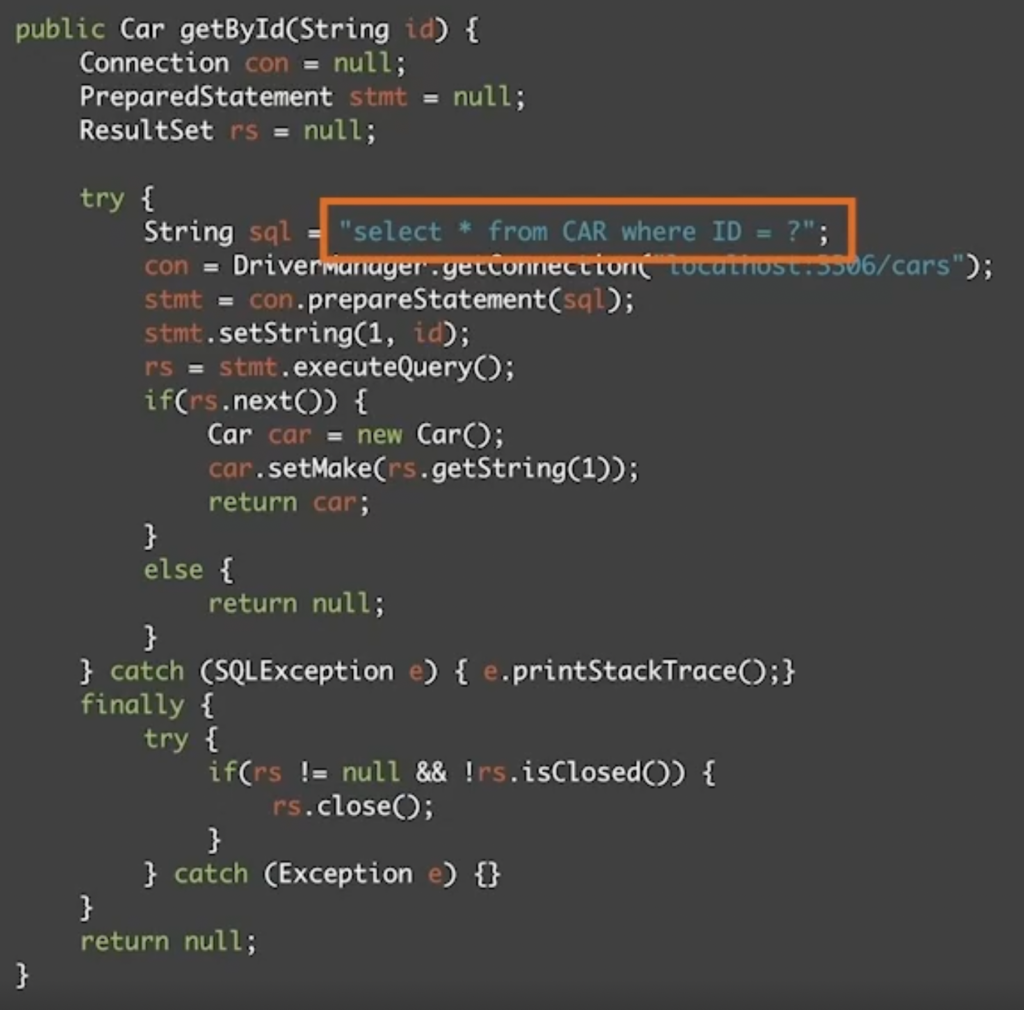
We only really care about two lines here:
- String sql = “select * from CAR where ID = ?”;
- car.setMake(rs.getString(1));
However, there is a lot of code that clutters up the space. Lucky for us, Spring can help with that by containing the places where we’re assigning, handling, and closing things. This is done by using Spring to inject these resources into our code.
The Solution
A good use of Spring is we can use it to remove configuration code or lookup code, and developers can focus on business needs. It can also create more testable code as things may be more hardcoded without Spring, as well as help us with annotation or XML-based development. This is all achieved through development through interfaces.
Business Focus Revisited
So earlier we saw how clunky a piece of code was written without Spring. Let’s take a look at what the code could look like with Spring.
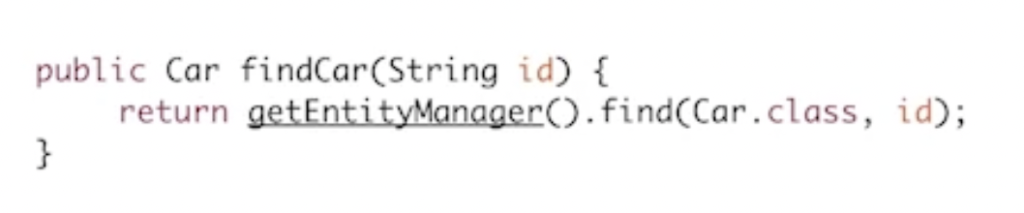
Yup, that’s it! There’s a black box that does everything we needed. We don’t have to worry about closing connections ourselves as Spring does everything for us.
How It Works
You can think of Spring as a glorified HashMap. It is called the “application context”, which is the configured Spring container with all the dependencies wired up in it.
Spring can also be used as a registry, which is how we’ll start it – with a main method and an application to run it. However, the main benefits of Spring will require us to go through its wiring constructs and use it to auto-wire our application.
Conclusion
So now that we’re done with this post, we’ve learned what the Spring framework is and how it is useful. Next, we’ll go over a few demos and learn about the architecture and project setup for Spring.